State Machine
A state machine is a model used to describe the transitions of an object between different states. It clearly shows how an object changes state based on events and may trigger corresponding actions.
Core Concepts
State: A distinct mode or condition of the system (e.g. “Idle”, “Running”). Managed by State class with optional on_enter/on_exit callbacks
Event: A trigger signal that may cause state transitions (e.g. “start”, “stop”)
Transition: A state change path from source to destination state triggered by an event
Action: An operation executed during transition (before entering new state)
Guard: A precondition that must be satisfied to allow transition
Code Link
- class MissionPlanning.StateMachine.state_machine.StateMachine(name: str, model=<class 'object'>)[source]
- __init__(name: str, model=<class 'object'>)[source]
Initialize the state machine.
- Parameters:
name (str) – Name of the state machine.
model (object, optional) – Model object used to automatically look up callback functions for states and transitions: State callbacks: Automatically searches for ‘on_enter_<state>’ and ‘on_exit_<state>’ methods. Transition callbacks: When action or guard parameters are strings, looks up corresponding methods in the model.
Example
>>> class MyModel: ... def on_enter_idle(self): ... print("Entering idle state") ... def on_exit_idle(self): ... print("Exiting idle state") ... def can_start(self): ... return True ... def on_start(self): ... print("Starting operation") >>> model = MyModel() >>> machine = StateMachine("my_machine", model)
- add_transition(src_state: str | State, event: str, dst_state: str | State, guard: str | Callable = None, action: str | Callable = None) None [source]
Add a transition to the state machine.
- Parameters:
src_state (str | State) – The source state where the transition begins. Can be either a state name or a State object.
event (str) – The event that triggers this transition.
dst_state (str | State) – The destination state where the transition ends. Can be either a state name or a State object.
guard (str | Callable, optional) – Guard condition for the transition. If callable: Function that returns bool. If str: Name of a method in the model class. If returns True: Transition proceeds. If returns False: Transition is skipped.
action (str | Callable, optional) – Action to execute during transition. If callable: Function to execute. If str: Name of a method in the model class. Executed after guard passes and before entering new state.
Example
>>> machine.add_transition( ... src_state="idle", ... event="start", ... dst_state="running", ... guard="can_start", ... action="on_start" ... )
- process(event: str) None [source]
Process an event in the state machine.
- Parameters:
event – Event name.
Example
>>> machine.process("start")
- register_state(state: str | State, on_enter=None, on_exit=None)[source]
Register a state in the state machine.
- Parameters:
state (str | State) – The state to register. Can be either a string (state name) or a State object.
on_enter (Callable, optional) – Callback function to be executed when entering the state. If state is a string and on_enter is None, it will look for a method named ‘on_enter_<state>’ in the model.
on_exit (Callable, optional) – Callback function to be executed when exiting the state. If state is a string and on_exit is None, it will look for a method named ‘on_exit_<state>’ in the model.
Example
>>> machine.register_state("idle", on_enter=on_enter_idle, on_exit=on_exit_idle) >>> machine.register_state(State("running", on_enter=on_enter_running, on_exit=on_exit_running))
PlantUML Support
The generate_plantuml()
method creates diagrams showing:
Current state (marked with [*] arrow)
All possible transitions
Guard conditions in [brackets]
Actions prefixed with /
Example
state machine diagram:
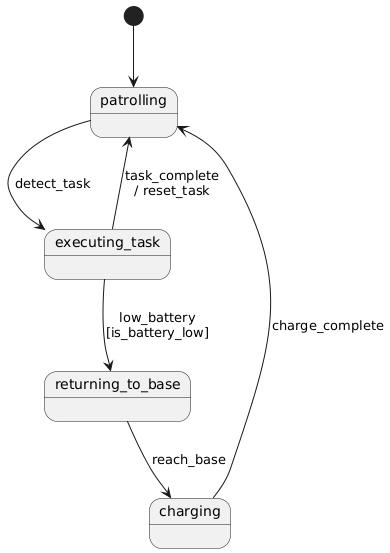
state transition table:
Source State |
Event |
Target State |
Guard |
Action |
---|---|---|---|---|
patrolling |
detect_task |
executing_task |
||
executing_task |
task_complete |
patrolling |
reset_task |
|
executing_task |
low_battery |
returning_to_base |
is_battery_low |
|
returning_to_base |
reach_base |
charging |
||
charging |
charge_complete |
patrolling |