Distance Map
This is an implementation of the Distance Map algorithm for path planning.
The Distance Map algorithm computes the unsigned distance field (UDF) and signed distance field (SDF) from a boolean field representing obstacles.
The UDF gives the distance from each point to the nearest obstacle. The SDF gives positive distances for points outside obstacles and negative distances for points inside obstacles.
Example
The algorithm is demonstrated on a simple 2D grid with obstacles:
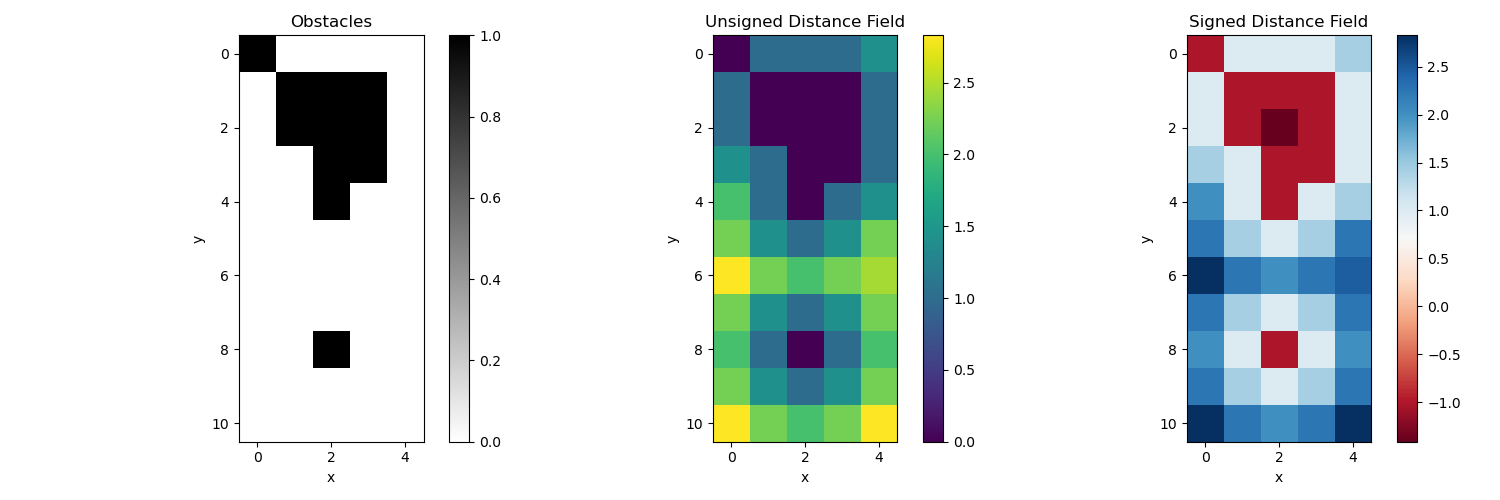
Code Link
- Mapping.DistanceMap.distance_map.compute_sdf(obstacles)[source]
Compute the signed distance field (SDF) from a boolean field.
- Parameters:
obstacles (array_like) – A 2D boolean array where ‘1’ represents obstacles and ‘0’ represents free space.
- Returns:
A 2D array representing the signed distance field, where positive values indicate distance to the nearest obstacle, and negative values indicate distance to the nearest free space.
- Return type:
array_like
- Mapping.DistanceMap.distance_map.compute_udf(obstacles)[source]
Compute the unsigned distance field (UDF) from a boolean field.
- Parameters:
obstacles (array_like) – A 2D boolean array where ‘1’ represents obstacles and ‘0’ represents free space.
- Returns:
A 2D array of distances from the nearest obstacle, with the same dimensions as bool_field.
- Return type:
array_like
References
Distance Transforms of Sampled Functions paper by Pedro F. Felzenszwalb and Daniel P. Huttenlocher.