Point cloud Sampling
This sections explains point cloud sampling algorithms in PythonRobotics.
Point clouds are two-dimensional and three-dimensional based data acquired by external sensors like LIDAR, cameras, etc. In general, Point Cloud data is very large in number of data. So, if you process all the data, computation time might become an issue.
Point cloud sampling is a technique for solving this computational complexity issue by extracting only representative point data and thinning the point cloud data without compromising the performance of processing using the point cloud data.
Voxel Point Sampling
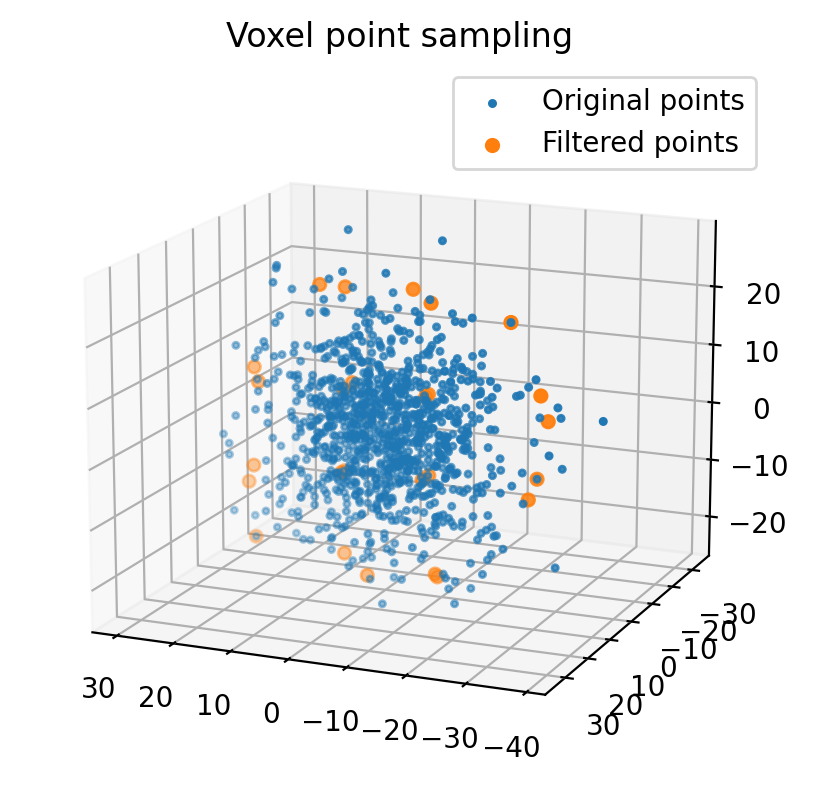
Voxel grid sampling is a method of reducing point cloud data by using the Voxel grids which is regular grids in three-dimensional space.
This method determines which each point is in a grid, and replaces the point clouds that are in the same Voxel with their average to reduce the number of points.
API
- Mapping.point_cloud_sampling.point_cloud_sampling.voxel_point_sampling(original_points: ndarray[tuple[int, ...], dtype[_ScalarType_co]], voxel_size: float)[source]
Voxel Point Sampling function. This function sample N-dimensional points with voxel grid. Points in a same voxel grid will be merged by mean operation for sampling.
- Parameters:
original_points ((M, N) N-dimensional points for sampling.) – The number of points is M.
voxel_size (voxel grid size) –
- Return type:
sampled points (M’, N)
Farthest Point Sampling
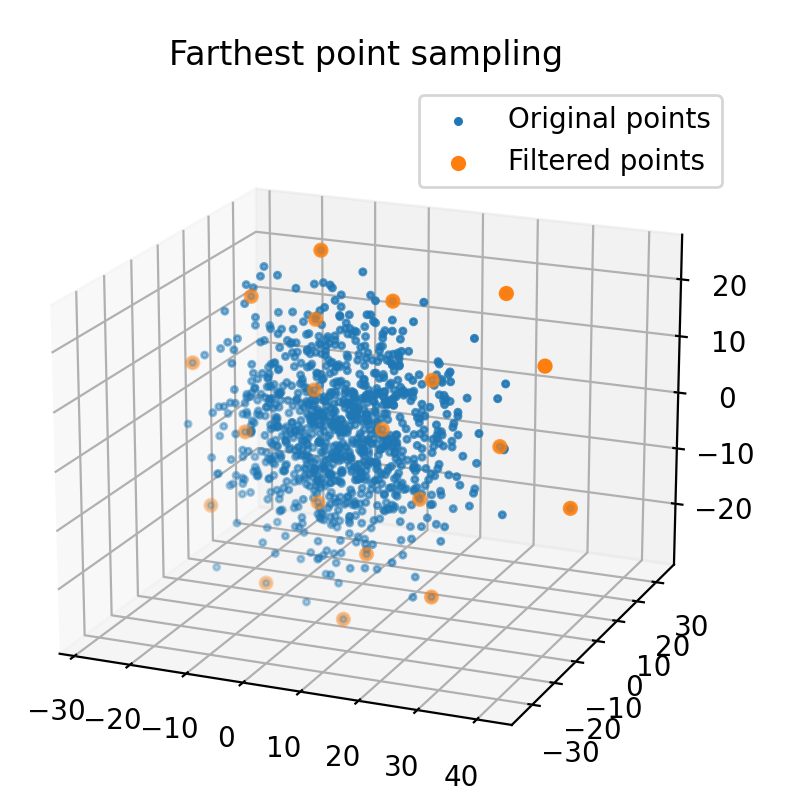
Farthest Point Sampling is a point cloud sampling method by a specified number of points so that the distance between points is as far from as possible.
This method is useful for machine learning and other situations where you want to obtain a specified number of points from point cloud.
API
- Mapping.point_cloud_sampling.point_cloud_sampling.farthest_point_sampling(orig_points: ndarray[tuple[int, ...], dtype[_ScalarType_co]], n_points: int, seed: int)[source]
Farthest point sampling function This function sample N-dimensional points with the farthest point policy.
- Parameters:
orig_points ((M, N) N-dimensional points for sampling.) – The number of points is M.
n_points (number of points for sampling) –
seed (random seed number) –
- Return type:
sampled points (n_points, N)
Poisson Disk Sampling
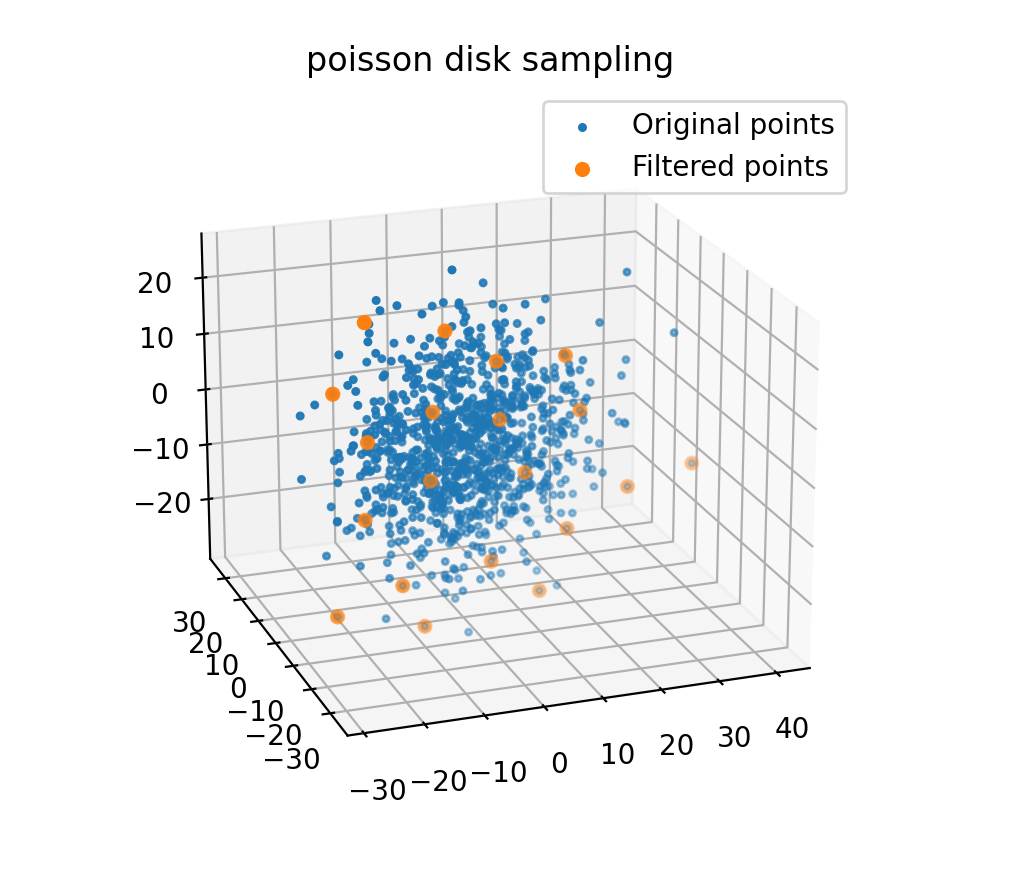
Poisson disk sample is a point cloud sampling method by a specified number of points so that the algorithm selects points where the distance from selected points is greater than a certain distance.
Although this method does not have good performance comparing the Farthest distance sample where each point is distributed farther from each other, this is suitable for real-time processing because of its fast computation time.
API
- Mapping.point_cloud_sampling.point_cloud_sampling.poisson_disk_sampling(orig_points: ndarray[tuple[int, ...], dtype[_ScalarType_co]], n_points: int, min_distance: float, seed: int, MAX_ITER=1000)[source]
Poisson disk sampling function This function sample N-dimensional points randomly until the number of points keeping minimum distance between selected points.
- Parameters:
orig_points ((M, N) N-dimensional points for sampling.) – The number of points is M.
n_points (number of points for sampling) –
min_distance (minimum distance between selected points.) –
seed (random seed number) –
MAX_ITER (Maximum number of iteration. Default is 1000.) –
- Return type:
sampled points (n_points or less, N)