Rapidly-Exploring Random Trees (RRT)
Basic RRT

This is a simple path planning code with Rapidly-Exploring Random Trees (RRT)
Black circles are obstacles, green line is a searched tree, red crosses are start and goal positions.
Code Link
RRT*

This is a path planning code with RRT*
Black circles are obstacles, green line is a searched tree, red crosses are start and goal positions.
Code Link
Simulation
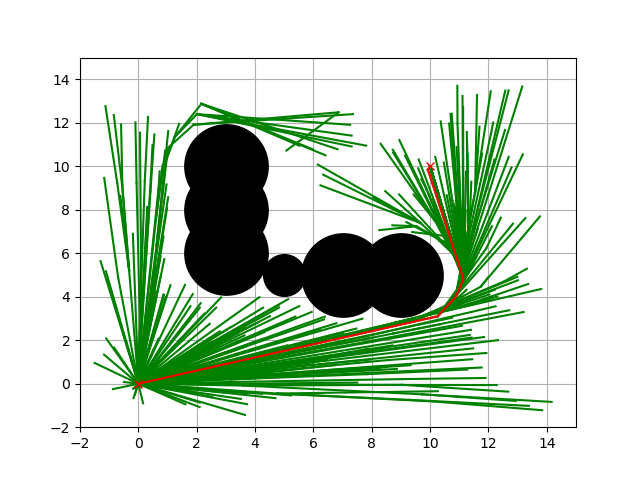
Ref
RRT with dubins path

Path planning for a car robot with RRT and dubins path planner.
Code Link
RRT* with dubins path

Path planning for a car robot with RRT* and dubins path planner.
Code Link
RRT* with reeds-sheep path

Path planning for a car robot with RRT* and reeds sheep path planner.
Code Link
Informed RRT*

This is a path planning code with Informed RRT*.
The cyan ellipse is the heuristic sampling domain of Informed RRT*.
Code Link
Reference
Batch Informed RRT*

This is a path planning code with Batch Informed RRT*.
Code Link
Reference
Closed Loop RRT*
A vehicle model based path planning with closed loop RRT*.

In this code, pure-pursuit algorithm is used for steering control,
PID is used for speed control.
Code Link
Reference
LQR-RRT*
This is a path planning simulation with LQR-RRT*.
A double integrator motion model is used for LQR local planner.
